(1)什么是进程和线程
(2)线程与进程的区别
(1)线程的六种状态
(2)线程状态验证
public static void main(String[] args) {new Thread(()->{try {System.in.read();} catch (IOException e) {e.printStackTrace();}}).start();
}
public static void main(String[] args) {Object obj = new Object();new Thread(()->{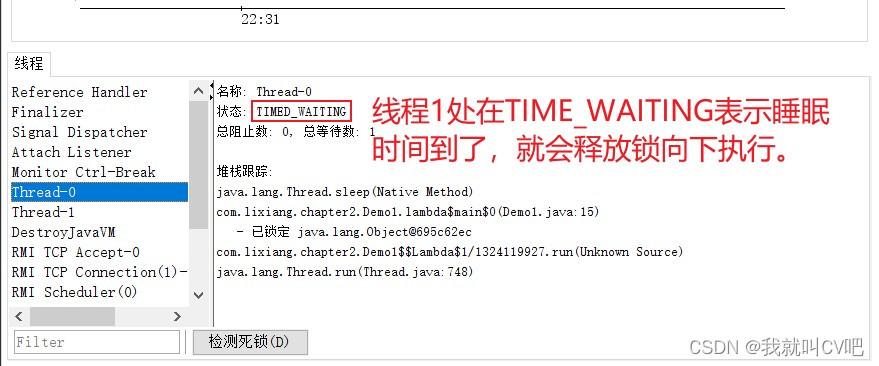synchronized (obj){try {//当线程拿到锁,无限睡眠,不释放锁System.out.println("线程1拿到锁");Thread.sleep(100000000L);} catch (InterruptedException e) {e.printStackTrace();}}}).start();new Thread(()->{synchronized (obj){//线程2模拟拿不到锁System.out.println("线程2拿到锁");}}).start();}
public static void main(String[] args) {Object obj = new Object();new Thread(()->{synchronized (obj){try {//获取锁对象调用wait()让其进入等待状态obj.wait();} catch (InterruptedException e) {e.printStackTrace();}}}).start();}
(3)线程的状态转换
(1)继承Thread类重写run()方法
public class MyThread extends Thread {@Overridepublic void run(){System.out.println("线程运行");}
}
public static void main(String[] args) {MyThread myThread = new MyThread();myThread.start();
}
(2)实现Runnable接口重写run()方法
public class MyRunnable implements Runnable{@Overridepublic void run() {System.out.println("线程运行");}
}
public static void main(String[] args) {new Thread(new MyRunnable()).start();
}
(3)实现Callable接口重写call()方法
public class MyCallable implements Callable {@Overridepublic Integer call() throws Exception {System.out.println("线程运行");return 1;}
}
public static void main(String[] args) {MyCallable callable = new MyCallable();FutureTask futureTask= new FutureTask<>(callable);new Thread(futureTask).start();
}
(1)什么是线程的挂起
(2)如何挂起线程
(3)suspend()、resume()案例
public class SuspendDemo implements Runnable{@Overridepublic void run() {System.out.println(Thread.currentThread().getName()+"执行run()方法,调用suspend()方法之前");/*** 线程挂起(废弃的方法)*/Thread.currentThread().suspend();System.out.println(Thread.currentThread().getName()+"执行run()方法,调用suspend()方法之后");}
}
public static void main(String[] args) throws InterruptedException {Thread thread = new Thread(new SuspendDemo());thread.start();/*** 对主线程进行休眠,以防目标线程先执行了唤醒操作,在执行挂起操作*/Thread.sleep(3000L);thread.resume();
}
(4)wait()、notify()案例
public class WaitDemo implements Runnable{private static Object object = new Object();@Overridepublic void run() {synchronized (object){try {System.out.println(Thread.currentThread().getName()+"获取锁资源");object.wait();System.out.println(Thread.currentThread().getName()+"执行业务逻辑");System.out.println(Thread.currentThread().getName()+"释放锁资源");} catch (InterruptedException e) {e.printStackTrace();}}}
}
public static void main(String[] args) throws InterruptedException {Thread thread1 = new Thread(new WaitDemo2(),"线程1");thread1.start();Thread.sleep(3000L);synchronized (object){object.notify();}
}
(1)stop()废弃方法,开发中不要使用,因为一旦调用,线程就会立刻停止,因此有可能会引发线程安全性问题。
public class InterruptedDemo extends Thread{private int i = 0;private int j = 0;@Overridepublic void run() {i++;try {Thread.sleep(2000L);} catch (InterruptedException e) {e.printStackTrace();}j++;}public void printf(){System.out.println("i:"+i);System.out.println("j:"+j);}
}
public static void main(String[] args) throws InterruptedException {InterruptedDemo thread1 = new InterruptedDemo();thread1.start();Thread.sleep(1000L);thread1.stop();thread1.printf();
}
(2)Thread.interrupt()方法,改变线程终止的标识,默认是false,线程执行,调用interrupt()方法标识改成true,线程停止执行。
public class InterruptedDemo extends Thread{@Overridepublic void run() {System.out.println("线程终止标识:"+Thread.currentThread().isInterrupted());while (!Thread.currentThread().isInterrupted()){System.out.println("业务逻辑执行");}System.out.println("线程终止标识:"+Thread.currentThread().isInterrupted());}
}
public static void main(String[] args) throws InterruptedException {InterruptedDemo thread1 = new InterruptedDemo();thread1.start();Thread.sleep(1);thread1.interrupt();
}
(3)自定义标识,通过判断来终止线程执行
public class InterruptedDemo extends Thread{//注意这里一定要加上volatile,防止指令重排private static volatile boolean FLAG = true;@Overridepublic void run() {System.out.println("线程执行标识:"+FLAG);while (FLAG){System.out.println("业务逻辑执行");}System.out.println("线程执行标识:"+FLAG);}
}
public static void main(String[] args) throws InterruptedException {InterruptedDemo thread1 = new InterruptedDemo();thread1.start();Thread.sleep(1);FLAG = false;
}
//setPrioity(num),设置最大优先级
thread.setPriority(Thread.MAX_PRIORITY);
public class PriorityDemo implements Runnable{@Overridepublic void run() {while(true){System.out.println(Thread.currentThread().getName()+":运行");}}public static void main(String[] args) {Thread thread1 = new Thread(new PriorityDemo(),"线程1");Thread thread2 = new Thread(new PriorityDemo(),"线程2");//设置线程1的优先级最大thread1.setPriority(Thread.MAX_PRIORITY);//设置线程2的优先级最小thread2.setPriority(Thread.MIN_PRIORITY);thread1.start();thread2.start();}}
public class DaemonDemo implements Runnable {@Overridepublic void run() {while(true){System.out.println(Thread.currentThread().getName());}}public static void main(String[] args) throws InterruptedException {Thread thread1 = new Thread(new DaemonDemo(),"线程1");//设置为守护线程thread1.setDaemon(true);thread1.start();Thread.sleep(2000L);}
}